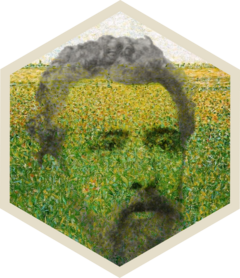
Run Scanorama on Seurat's Assay5 object through IntegrateLayers
Source: R/Scanorama.R
ScanoramaIntegration.Rd
A wrapper to run Scanorama
on multi-layered Seurat V5 object.
Requires a conda environment with scanorama
and necessary dependencies
Usage
ScanoramaIntegration(
object,
orig,
layers = NULL,
features = NULL,
scale.layer = "scale.data",
conda_env = NULL,
new.reduction = "integrated.scanorama",
reduction.key = "scanorama_",
reconstructed.assay = "scanorama.reconstructed",
ncores = 1L,
ndims.out = 100L,
return_dense = TRUE,
batch_size = 5000,
approx = TRUE,
sigma = 15L,
alpha = 0.1,
knn = 20L,
hvg.scanorama = NULL,
union.features = FALSE,
sketch = FALSE,
sketch_method = c("geosketch", "uniform"),
sketch_max = 10000,
seed.use = 42L,
verbose = TRUE,
...
)
Arguments
- object
A
Seurat
object (or anAssay5
object if not called byIntegrateLayers
)- orig
DimReduc
object. Not to be set directly when called withIntegrateLayers
, useorig.reduction
argument instead- layers
Name of the layers to use in the integration
- features
Vector of feature names to input to the integration method. When
features = NULL
(default), theVariableFeatures
are used. To pass all features, use the output ofFeatures()
- scale.layer
Name of the scaled layer in
Assay
- conda_env
Path to conda environment to run scanorama (should also contain the scipy python module). By default, uses the conda environment registered for scanorama in the conda environment manager
- new.reduction
Name to store resulting dimensional reduction object.
NULL
orFALSE
disables the dimension reduction computation- reduction.key
Key for resulting dimensional reduction object. Ignored if
reduction.key
isNULL
orFALSE
- reconstructed.assay
Name for the
assay
containing the corrected expression matrix of dimension #features
x #cells.NULL
orFALSE
disables the corrected expression matrix computation- ncores
Number of parallel threads to create through blas_set_num_threads. Pointless if BLAS is not supporting multithreaded
- ndims.out
Number of dimensions for
new.reduction
output. Scanorama specific argument- return_dense
Whether scanorama returns
numpy.ndarray
matrices instead ofscipy.sparse.csr_matrix
. Scanorama specific argument- batch_size
Used in the alignment vector computation. Higer values increase memory burden.Scanorama specific argument
- approx
Whether scanorama approximates nearest neighbors computation. Scanorama specific argument
- sigma
Correction smoothing parameter on gaussian kernel. Scanorama specific argument
- alpha
Minimum alignment score. Scanorama specific argument
- knn
Number of nearest neighbours used for matching. Scanorama specific argument
- hvg.scanorama
A positive integer to turn scanorama's internal HVG selection on. Disabled by default. Scanorama specific argument
- union.features
By default, scanorama uses the intersection of features to perform integration. Set this parameter to
TRUE
to use the union. Discouraged. Scanorama specific argument- sketch
Turns on sketching-based acceleration by first downsampling the datasets. See Hie et al., Cell Systems (2019). Disabled by default. Ignored when
reconstructed.assay
is enabled. Scanorama specific argument- sketch_method
A skething method to apply to the data. Either 'geosketch' (default) or 'uniform'. Ignored when
reconstructed.assay
is enabled or whensketch
is FALSE. Scanorama specific argument- sketch_max
Downsampling cutoff. Ignored when sketching disabled. Scanorama specific argument
- seed.use
An integer to generate reproducible outputs. Set
seed.use = NULL
to disable- verbose
Print messages. Set to
FALSE
to disable- ...
Ignored
Value
A list containing at least one of:
a new DimReduc of name
reduction.name
(key set toreduction.key
) with corrected embeddings matrix ofndims.out
.a new Assay of name
reconstructed.assay
with corrected counts for eachfeatures
(or less ifhvg
is set to a lower integer).
When called via IntegrateLayers
, a Seurat object with
the new reduction and/or assay is returned
Note
This function requires the Scanorama package to be installed (along with scipy)
References
Hie, B., Bryson, B. & Berger, B. Efficient integration of heterogeneous single-cell transcriptomes using Scanorama. Nat Biotechnol 37, 685–691 (2019). DOI
Examples
if (FALSE) { # \dontrun{
# Preprocessing
obj <- SeuratData::LoadData("pbmcsca")
obj[["RNA"]] <- split(obj[["RNA"]], f = obj$Method)
obj <- NormalizeData(obj)
obj <- FindVariableFeatures(obj)
obj <- ScaleData(obj)
obj <- RunPCA(obj)
# After preprocessing, we integrate layers:
obj <- IntegrateLayers(object = obj, method = ScanoramaIntegration)
# To disable feature expression matrix correction:
obj <- IntegrateLayers(object = obj, method = ScanoramaIntegration,
reconstructed.assay = NULL)
} # }